ExcelからSVGを生成
Excelファイルを元データにして、SVGを出力するプログラムを作ってみました。
下図のように16×16マスの正方形を塗りつぶしたExcelファイルをアップロードすると、その内容のプレビューとSVGを出力します。
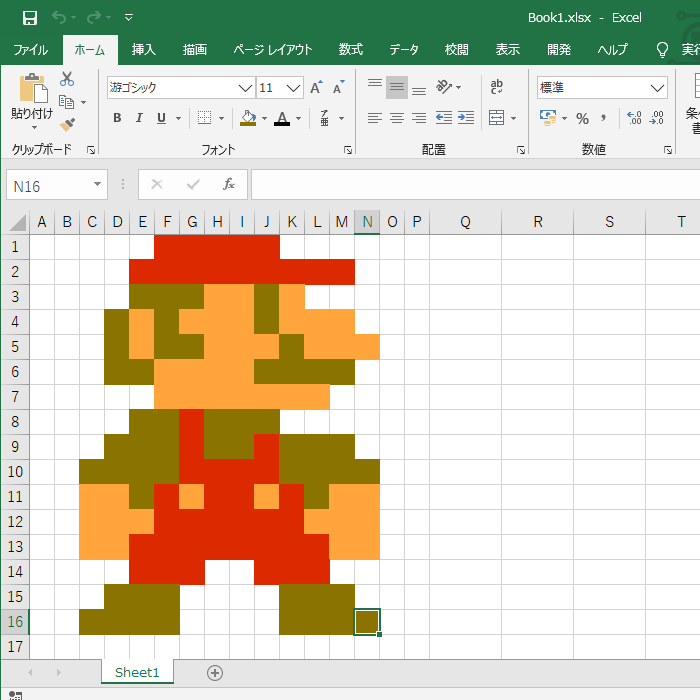
使い方はこんな感じ
実は『世界樹の迷宮』のマップを作るために作成したマップ生成ツールは、この技術の応用なんですね。
ソースコードを公開しておくので、役立ててくだされば幸いです。
■ソースコード(PHP Ver7.1, PhpSpreadsheet Ver1.2.1で動作を確認)
※ |
PhpSpreadsheet Ver1.2.1はPHP Ver7.3では動作しませんでした。 |
index.php
<?php
require_once('vendor/autoload.php');
use PhpOffice\PhpSpreadsheet\Reader\Xlsx;
use PhpOffice\PhpSpreadsheet\Style\Fill;
function getCellColor($cell) {
$style = $cell->getStyle();
if (empty($style)) {
return null;
}
$fill = $style->getFill();
if (empty($fill)) {
return null;
}
if ($fill->getFillType() === Fill::FILL_NONE) {
return null;
}
$color = $fill->getStartColor();
if (empty($color)) {
return null;
}
$argb = $color->getARGB();
if (substr($argb, 0, 2) === '00') {
return null;
}
return $color->getRGB();
}
$svg = null;
if (!empty($_FILES['file_name']['tmp_name'])) {
$tmp = $_FILES['file_name']['tmp_name'];
$reader = new Xlsx();
$book = $reader->load($tmp);
$sheet = $book->getSheet(0);
$svg = '<svg xmlns="http://www.w3.org/2000/svg" width="' . (18 * 16) . '" height="' . (18 * 16) . '" version="1.1">' . PHP_EOL;
for($row = 0; $row < 16; $row++){
for($column = 0; $column < 16; $column++){
$cell = $sheet->getCellByColumnAndRow($column + 1, $row + 1);
$color = getCellColor($cell);
if($color != null){
$svg .= ' <rect x="' . (18 * $column) . '" y="' . (18 * $row) . '" width="18" height="18" stroke="none" fill="#' . $color . '" />' . PHP_EOL;
}
}
}
$svg .= '</svg>' . PHP_EOL;
}
?>
<!DOCTYPE html>
<html>
<body>
<form action="./index.php" method="post" enctype="multipart/form-data">
<table>
<tr>
<td><input type="file" name="file_name" accept=".xlsx,application/msexcel" required /></td>
<td style="text-align:left; width:100%;"><input type="submit" value="Upload" /></td>
</tr>
<tr>
<td>Preview</td>
</tr>
<tr>
<td colspan="2">
<div style="width:288px; height:288px; background-color:#f0f0f0">
<?php echo (isset($svg) ? $svg : ''); ?>
</div>
</td>
</tr>
<tr>
<td>HTML</td>
</tr>
<tr>
<td colspan="2">
<textarea style="width:720px; height:300px; background-color:black; color:white;">
<?php echo (isset($svg) ? $svg : ''); ?>
</textarea>
</td>
</tr>
</table>
</form>
</body>
</html>
戻る